Transformation in Apache spark
Transformation in Apache Spark is a function that can be applied to an R.D.D. The output of a transformation is another R.D.D.
A transformation does not change the input R.D.D. We can also create a pipeline of certain transformations to create a data flow.
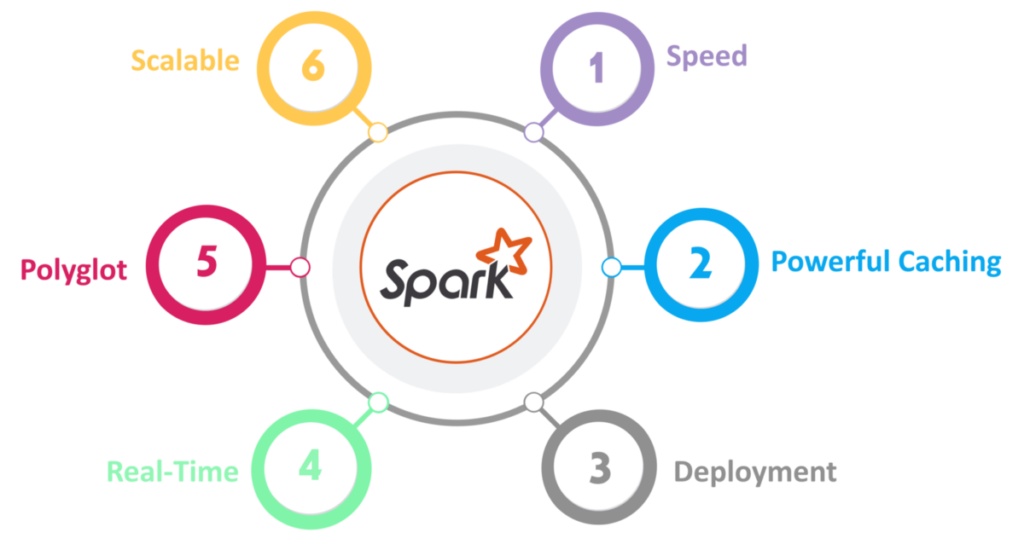